Poker Odds Calculator Python
- Reminds me of the 2+2 poker hand evaluator - they basically built an insanely fast giant lookup table that fit in 100megs of ram IIRC. The main idea was to do monte-carlo simulations and give you your odds of winning at any point in the game by dealing random cards for the unknowns. Interesting read on that and other evaluators as well.
- Poker odds calculate the chances of you holding a winning hand. The poker odds calculators on CardPlayer.com let you run any scenario that you see at the poker table, see your odds and outs,.
- PokerListings.com’s Poker Odds Calculator is the fastest, most accurate and easy-to-use poker odds calculator online. It’s just like what you see when you watch poker on TV. Use it in real-time to know exactly what your chances of winning and losing are at any point in a poker hand – be it on online poker sites or playing live poker.
Poker Variance Calculator This variance calculator and simulator for poker is handy and easy to use. Just enter your winrate, standard deviation and the amount of hands to simulate. You'll most certainly get insightful results.Read below how to use this simulator. The Poker Odds Calculator by Cardschat is a free mobile app that’s simple, easy to use and about as straight forward as it gets for a poker equity calculator. You can calculate the odds of winning for anywhere from 2-10 players for Texas Hold’em, and also dive into calculations for poker variants like Omaha, Razz and Seven Card Stud.
Released:
Holdem Calculator library
Project description
The Holdem Calculator library calculates the probability that a certain Texas Hold'em hand will win. This probability is approximated by running a Monte Carlo method or calculated exactly by simulating the set of all possible hands. The Holdem Calculator also shows how likely each set of hole cards is to make a certain poker hand. The default Monte Carlo simulations are generally accurate to the nearest percent. Accuracy can be improved by increasing the number of simulations that are run, but this will result in a longer running time.
Command Line Options
Usage
I've listed a few examples showing how to use the Holdem Calculator. Note that you can mix and match command line options to suit your needs. See the bottom example in this section to see how to use the multiprocess Holdem Calculator for faster computations.
Default use case:
Multiplayer use case:
Exact calculation:
Board supplied:
Input file supplied:
In order to calculate multiple hands in a single run, the user has the choice to allow Holdem Calculator to read from an input file. Each line of the input file should represent a single calculation. Hole cards and boards should be separated using a ' ' divider.
Unknown Hole Cards:
Compute how likely a hand is to win against a random pair of hole cards. You can only specify one set of hole cards as unknown.Note: Performing calculations with unknown hole cards takes an excessively long time if community cards are not specified.
Multiprocess Holdem Calculator:
Takes the same command line options but utilizes multicore processors to increase the speed of computation.Windows users: Due to the process forking mechanism in Windows, parallel_holdem_calc might be slower than expected.
Library Calls:
If you want to use Holdem Calculator as a library, you can import holdem_calc or parallel_holdem_calc and call calculate(). The order of arguments to calculate() are as follows:
- Board: These are the community cards supplied to the calculation. This is in the form of a list of strings, with each string representing a card. If you do not want to specify community cards, you can set board to be None. Example: ['As', 'Ks', 'Jd']
- Exact: This is a boolean which is True if you want an exact calculation, and False if you want a Monte Carlo simulation.
- Number of Simulations: This is the number of iterations run in the Monte Carlo simulation. Note that this parameter is ignored if Exact is set to True. This number must be positive, even if Exact is set to true.
- Input File: The name of the input file you want Holdem Calculator to read from. Mark as None, if you do not wish to read from a file. If Input File is set, library calls will not return anything.
- Hole Cards: These are the hole cards for each of the players. This is in the form of a list of strings, with each string representing a card. Example: ['As', 'Ks', 'Jd', 'Td']
- Verbose: This is a boolean which is True if you want Holdem Calculator to print the results.
Calls to calculate() return a list of floats. The first element in the list corresponds to the probability that a tie takes place. Each element after that corresponds to the probability one of the hole cards the user provides wins the hand. These probabilities occur in the order in which you list them.
Poker Odds Calculator Python Calculator
Copyright
Copyright (c) 2013 Kevin Tseng. See LICENSE for details.
Release historyRelease notifications RSS feed
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Filename, size | File type | Python version | Upload date | Hashes |
---|---|---|---|---|
Filename, size holdem_calc-1.0.0-py3-none-any.whl (14.4 kB) | File type Wheel | Python version py3 | Upload date | Hashes |
Filename, size holdem_calc-1.0.0.tar.gz (15.3 kB) | File type Source | Python version None | Upload date | Hashes |
Hashes for holdem_calc-1.0.0-py3-none-any.whl
Algorithm | Hash digest |
---|---|
SHA256 | b153aeaf57db68efb42b801d617823cddd07898af777a497309cc5874df6cfd1 |
MD5 | c93a59c622217c026573083492626827 |
BLAKE2-256 | ecc5ea3ed2f22ff936a22d9f983ff590e72252e4d505c04fcc7f0358502476e9 |
Hashes for holdem_calc-1.0.0.tar.gz
Algorithm | Hash digest |
---|---|
SHA256 | 28a124902c0b247b8651e326df680c488171325b212aeeb8809bed0d30d8f06a |
MD5 | 46fab1f2d4de43e725a69bb67d2f4500 |
BLAKE2-256 | 4520eeb82fb1d1470f32d97b386cef005a785c6c168b3057336cb29baf8eb736 |
Hey guys,
If you’re an avid poker player, or even if you’re just starting out, I invite you to check out the poker odds calculator for Texas Hold ’em. With this tool, you can calculate your odds for winning any game. I found a very polished calculator that is available here.
Basic operation of a calculator is adding the cards to your hand and community. It then determines the odds your hand has of winning.
In this article, I’ll cover how you can code a simple poker odds calculator. First, let’s go over how the calculator stores the cards in your hand and the community.
Setup Card Data
A card is the basic data block we’ll be using. We’ll create a simple Card
object, that will store the suit and value of each card. You can write it as:
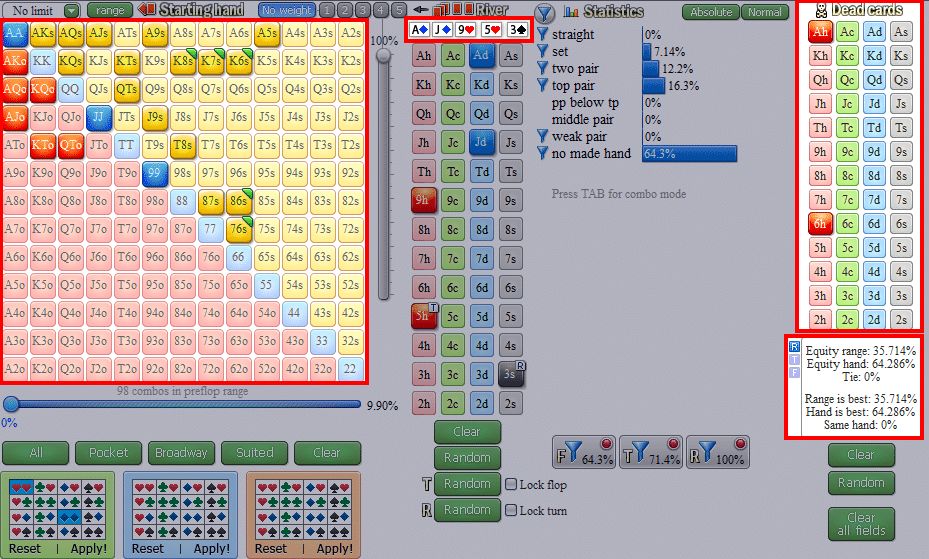
A player’s hand is stored as an array of Card
objects. The array has two elements, one for each card. You’d setup that up like this:
Poker Odds Calculator Python Match
Note: If you’d like to see, in detail, the setup for card values, suits, Card
objects, and hands, please see this link to an earlier article.
Setup Player and Community Data
The player’s hand and community cards are both defined as arrays, and will contain several Card
objects:
Adding Player And Community Cards To Be Played
To add a card to the player’s hand, construct a new Card
object, set its value and suit, then add it to the playerHand
array. Say you want to add the 4♣. You’d write:
Adding a community card is similar. To add a Q♦:
Evaluating Odds
Now that you have set up the players and cards, you can begin calculating odds.
First, create the scenario you want to evaluate. In the following example, we’ll use four community cards from the flop and turn. Let’s say your hand is 4♣ 9♣, and the community cards are 7♦, 5♣ , K♥, 8♣. You’d code this as:
Then, determine the best possible hand for the player using the cards in the player’s hand, and the community cards. You do this by finding how many “outs” you have. An out is an unknown card (in this case, the final river card) that, when added, will give you the best hand.
Next, determine which potential river cards would give you a strong or winning hand (a flush or straight): any 6 (four cards), or 2♣, 3♣, 7♣, 10♣, J♣, Q♣, K♣, and A♣. So, there are a total of 12 outs.
Note: Writing code to determine the outs themselves, or compare odds for multiple players both go beyond the scope of this article. I may cover them in future articles.
When matching and comparing poker hands, you may find it easier to use a “temporary hand” (a new array) when evaluating numerous combinations of the player’s cards with the community cards. For example, consider this:

The above code gives you a working hand of 9♣, 7♦, 5♣, K♥, 8♣. You could then perform the various card matching actions on this array, using functions from previous articles, such as sorting ( CardMatchUtils.SortCardsByDescendingValue
), or determining if a hand is a straight ( CardMatchUtils.AreCardsStraight
).
To calculate the percentage you have of getting one of these out cards, you need to factor in the remaining number of cards in the deck, which is 46. A breakdown is:
Of those 46 cards, 12 of them (the outs we found earlier) will give you a strong or winning hand. You can find the percentage of getting one of them with this:
In our example, this is 1 – (46 – 12) / 46 = 0.26, or a 26% chance.
If you want to go further and represent this in odds notation (“x to y”), do this calculation:
Which yields, 1 / 0.26 – 1 = 2.8, or “2.8 : 1”. This means for about every 3 games, you might get a flush or straight.
That’s it for this article! I’ll talk to you next time. Thanks and take care 🙂
– C. out.